This post is w.r.t the post where in I had consumed WCF Data service in an android application. I have used SQL Server 2012 database, visual studio 2012 using ADO.Net
entity framework for creation of dataservices. We will create a sample WCF data service over an adventureworks database, this database can be downloaded from the
following link - Download.
Step1 - In visual studio 2012 click on New Projects on the start page and click on ASP.NET web forms as shown in the image below.
Step2 - Rightclick on the project and create a new folder and name it as AdventureDWData
Step3 - Once this is done, right click on the folder and click on new item. In the selection search for ADO.Net Entity Framework and name it as adventureModel.edmx
Step4 - It will give you an option to generate the entity model based on existing database or you can create your own tables in this which will inturn be made into a
database by the layer. Here we choose generate from database and click on next
Step5 - Click on New Connections and provide the servername and credentials as shown in the image below Save the webconfig as AdventureWorksDW2012Entities1 and click
on next.
Step6 - Then you will be taken to a screen where in you can select the list of table / views / stored procedures which you would like to expose in the data service. I
will go ahead and select all the tables as shown in the image below and click on Finish.
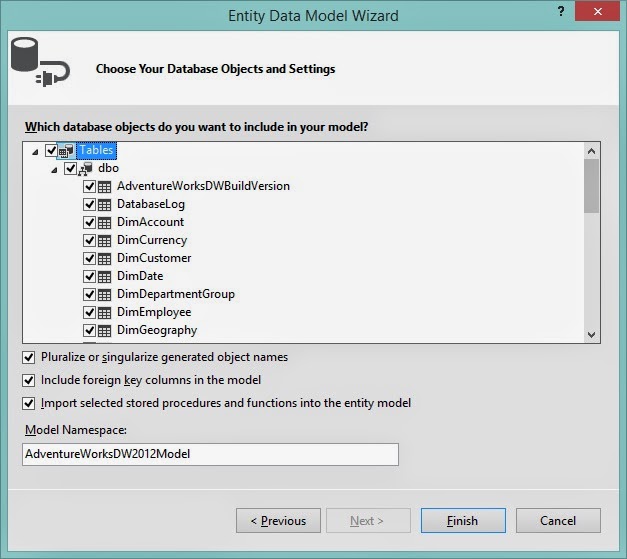
Step7 - Once done you can see the tables which have been pulled into the entity model which is shown in the image below.
Now that we have created the entity model with the list of objects to be exposed in the service let us go ahead and create the data service
Step8 - Right click on the solution and click on add > new item. In the selection click on WCF Data Service and name it AdventureDS1.
Step9 - Once done you will be taken to a AdventureDS.svc.cs, this where you bind the dataservice with the entity model. We need to do the following modifications
a) Tell the dataservice where your entity model is stored by -
"using AdventureworksDataService.AdventureDWData;"
b) You need to tell the dataservice is over the following entitymodel this is done using the code below
public class AdventureDS : DataService< AdventureWorksDW2012Entities >
c) In the Initialize service function you can find the line "//config.SetEntitySetAccessRule("MyEntityset", EntitySetRights.AllRead);". Uncomment this line
and modify it as "config.SetEntitySetAccessRule("*", EntitySetRights.AllRead);"
This is where you go ahead and mention the accessrights you want to set on each table / sp / views in your entitymodel. Here I have mentioned it as * which
means that all the entities in the entity model are readonly.
The final code will like below:
using System;
using System.Collections.Generic;
using System.Data.Services;
using System.Data.Services.Common;
using System.Linq;
using System.ServiceModel.Web;
using System.Web;
using AdventureworksDataService.AdventureDWData;
namespace AdventureworksDataService
{
public class AdventureDS : DataService< AdventureWorksDW2012Entities1 >
{
// This method is called only once to initialize service-wide policies.
public static void InitializeService(DataServiceConfiguration config)
{
// TODO: set rules to indicate which entity sets and service operations are visible, updatable, etc.
// Examples:
config.SetEntitySetAccessRule("*", EntitySetRights.AllRead);
// config.SetServiceOperationAccessRule("MyServiceOperation", ServiceOperationRights.All);
config.DataServiceBehavior.MaxProtocolVersion = DataServiceProtocolVersion.V3;
}
}
}
Step 10 - Right click on the service and select set as startpage.
Now right click on the solution and run it and you can see the following image as shown below with the url http://localhost:26636/AdventureDS1.svc/
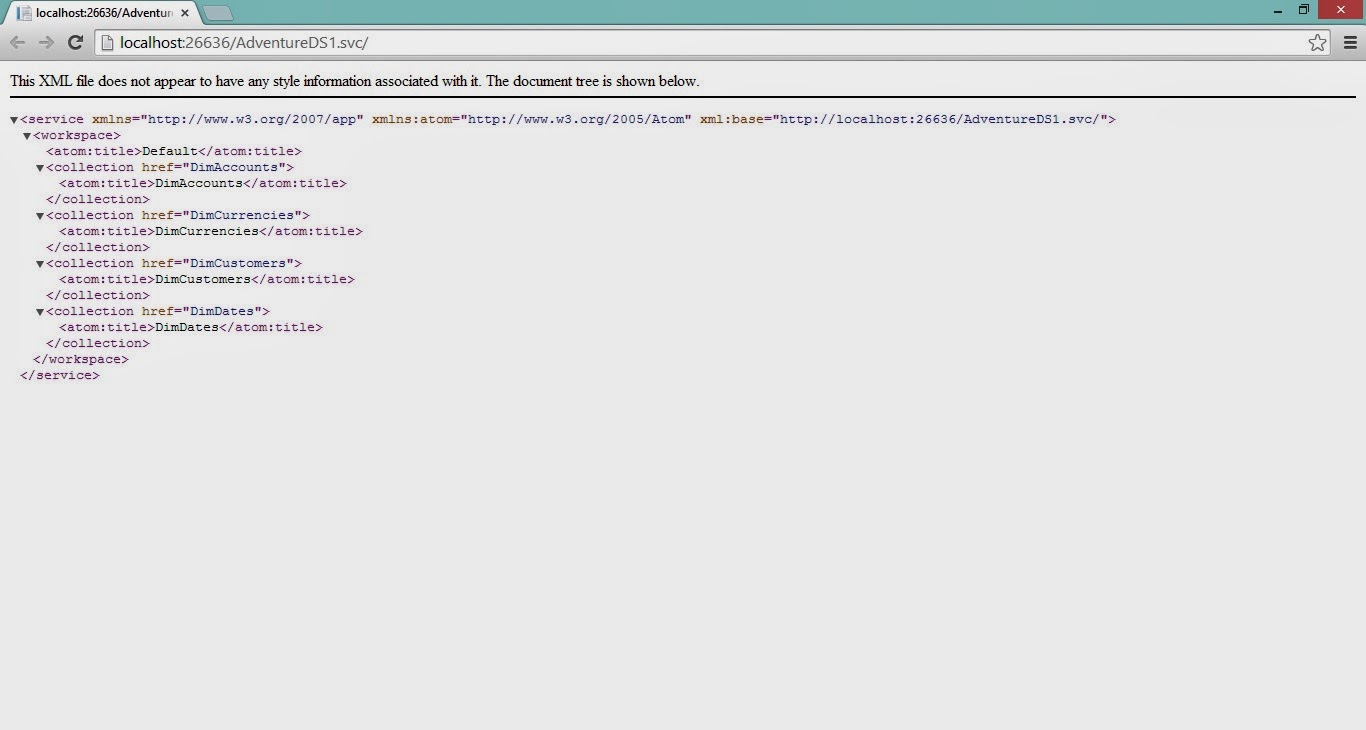
If you add http://localhost:26636/AdventureDS1.svc/$metadata you can see the list of all the table and columns exposed in the data service as shown in the image below.
entity framework for creation of dataservices. We will create a sample WCF data service over an adventureworks database, this database can be downloaded from the
following link - Download.
Step1 - In visual studio 2012 click on New Projects on the start page and click on ASP.NET web forms as shown in the image below.
Step2 - Rightclick on the project and create a new folder and name it as AdventureDWData
Step3 - Once this is done, right click on the folder and click on new item. In the selection search for ADO.Net Entity Framework and name it as adventureModel.edmx
Step4 - It will give you an option to generate the entity model based on existing database or you can create your own tables in this which will inturn be made into a
database by the layer. Here we choose generate from database and click on next
Step5 - Click on New Connections and provide the servername and credentials as shown in the image below Save the webconfig as AdventureWorksDW2012Entities1 and click
on next.
Step6 - Then you will be taken to a screen where in you can select the list of table / views / stored procedures which you would like to expose in the data service. I
will go ahead and select all the tables as shown in the image below and click on Finish.
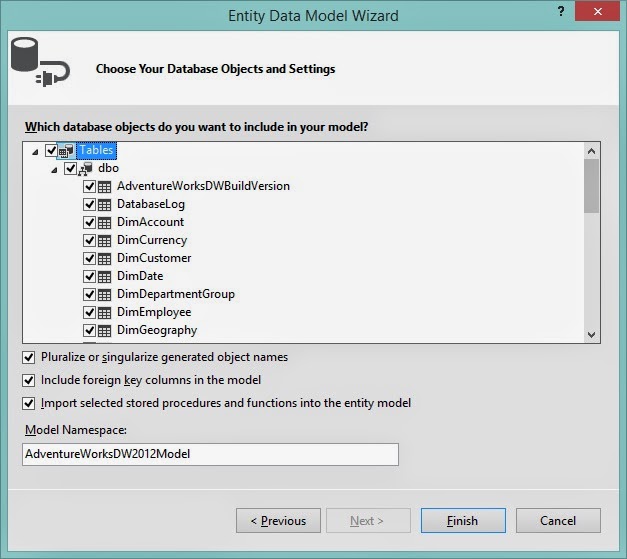
Step7 - Once done you can see the tables which have been pulled into the entity model which is shown in the image below.
Now that we have created the entity model with the list of objects to be exposed in the service let us go ahead and create the data service
Step8 - Right click on the solution and click on add > new item. In the selection click on WCF Data Service and name it AdventureDS1.
Step9 - Once done you will be taken to a AdventureDS.svc.cs, this where you bind the dataservice with the entity model. We need to do the following modifications
a) Tell the dataservice where your entity model is stored by -
"using AdventureworksDataService.AdventureDWData;"
b) You need to tell the dataservice is over the following entitymodel this is done using the code below
public class AdventureDS : DataService< AdventureWorksDW2012Entities >
c) In the Initialize service function you can find the line "//config.SetEntitySetAccessRule("MyEntityset", EntitySetRights.AllRead);". Uncomment this line
and modify it as "config.SetEntitySetAccessRule("*", EntitySetRights.AllRead);"
This is where you go ahead and mention the accessrights you want to set on each table / sp / views in your entitymodel. Here I have mentioned it as * which
means that all the entities in the entity model are readonly.
The final code will like below:
using System;
using System.Collections.Generic;
using System.Data.Services;
using System.Data.Services.Common;
using System.Linq;
using System.ServiceModel.Web;
using System.Web;
using AdventureworksDataService.AdventureDWData;
namespace AdventureworksDataService
{
public class AdventureDS : DataService< AdventureWorksDW2012Entities1 >
{
// This method is called only once to initialize service-wide policies.
public static void InitializeService(DataServiceConfiguration config)
{
// TODO: set rules to indicate which entity sets and service operations are visible, updatable, etc.
// Examples:
config.SetEntitySetAccessRule("*", EntitySetRights.AllRead);
// config.SetServiceOperationAccessRule("MyServiceOperation", ServiceOperationRights.All);
config.DataServiceBehavior.MaxProtocolVersion = DataServiceProtocolVersion.V3;
}
}
}
Step 10 - Right click on the service and select set as startpage.
Now right click on the solution and run it and you can see the following image as shown below with the url http://localhost:26636/AdventureDS1.svc/
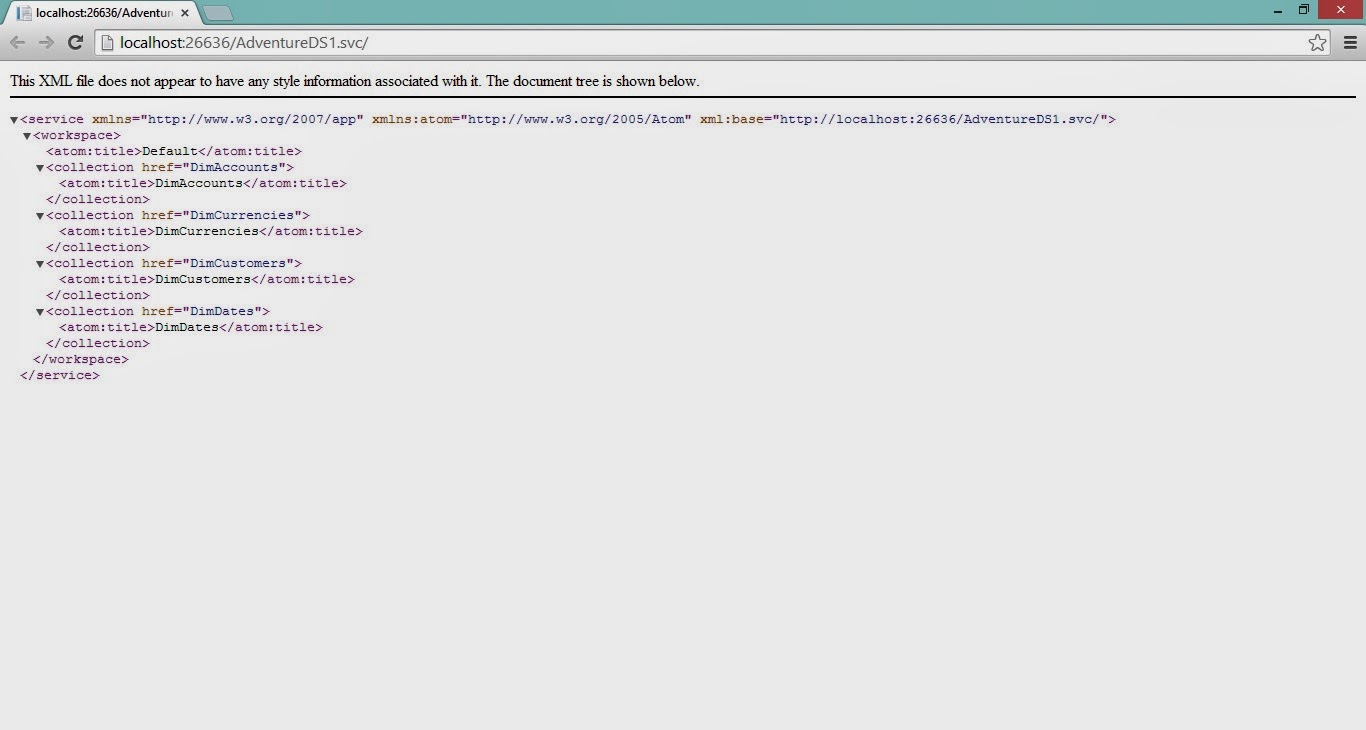
If you add http://localhost:26636/AdventureDS1.svc/$metadata you can see the list of all the table and columns exposed in the data service as shown in the image below.